The status of running process is the name of the current scope the process is in. To test the Java program creat a BPEL process with some scopes. Each scope is waiting of a few minutes. Running the Java program multiple times, results that you see that the status of the process is chaning.
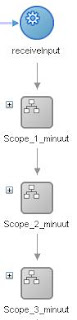
The program:
RMIListInstances.java
package nl.orasoa.bpel;
import com.collaxa.xml.XMLHelper;
import com.oracle.bpel.client.BPELProcessId;
import com.oracle.bpel.client.IBPELProcessHandle;
import com.oracle.bpel.client.IInstanceHandle;
import com.oracle.bpel.client.Locator;
import com.oracle.bpel.client.NormalizedMessage;
import com.oracle.bpel.client.delivery.IDeliveryService;
import com.oracle.bpel.client.util.WhereCondition;
import com.oracle.bpel.client.util.WhereConditionHelper;
import java.util.Map;
import java.util.Properties;
import org.w3c.dom.Element;
public class RMIListInstances
{
public static void main(String[] args)
throws Exception
{
IInstanceHandle[] instHandles;
IBPELProcessHandle[] procHandles;
IInstanceHandle instance = null;
BPELProcessId bpid = null;
WhereCondition cond;
WhereCondition cond1;
WhereCondition cond2;
try
{
// properties in the classpath
Properties props = new java.util.Properties();
// read the properties file
java.net.URL url =
ClassLoader.getSystemResource("context.properties");
props.load(url.openStream());
System.out.println(props);
// WhereCondition cond =
// WhereConditionHelper.whereInstancesClosed();
// WhereCondition cond =
// WhereConditionHelper.whereInstancesCompleted();
// Search only open instances
cond = WhereConditionHelper.whereInstancesOpen();
// append where clause to search for
// a specific process and version
cond1 = new WhereCondition( "process_id = ?" );
cond1.setString(1, "Wacht");
cond2 = new WhereCondition( "revision_tag = ?" );
cond2.setString(1, "1.0");
// append the whole where clause
cond.append("and").append(cond1).append("and").append(cond2);
// get a connection to the server
Locator locator = new Locator("default", "welcome1", props);
instHandles = locator.listInstances(cond);
for (int j = 0; j < instHandles.length; j++)
{
// get the instance
instance = instHandles[j];
// get the process for the instance
bpid = instance.getProcess().getProcessId();
System.out.print("Got process " + bpid.getProcessId()
+ "("+ bpid.getRevisionTag() + ") ");
System.out.println(instance.getConversationId() + " "
+ instance.getStatus() + " " + instance.getTitle());
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}