The reason why I wrote this example, is that I did not find a straight forward simple java program to do this. Examples are supplied with BPEL (no 102) but not exactly what I want.
To run the example, open in JDeveloper a new project and add the following java class into your project. Make sure you add the following libraries to your project to make to java program runnable.
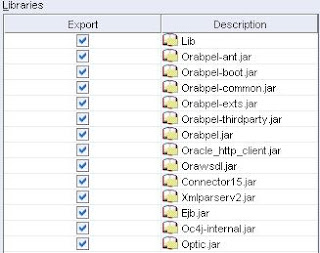
context.properties
orabpel.platform=ias_10g
java.naming.factory.initial=com.evermind.server.rmi.RMIInitialContextFactory
java.naming.provider.url=opmn:ormi://edison.eurotransplant.nl:6003:oc4j_soa/orabpel
java.naming.security.principal=oc4jadmin
java.naming.security.credentials=scanner1
Here is the class file.
RMIClient.java
package nl.orasoa.bpel;
import com.collaxa.xml.XMLHelper;
import com.oracle.bpel.client.Locator;
import com.oracle.bpel.client.NormalizedMessage;
import com.oracle.bpel.client.delivery.IDeliveryService;
import java.util.Map;
import java.util.Properties;
import org.w3c.dom.Element;
public class RMIClient
{
public static
void main(String[] args)
throws Exception
{
try
{
String who = "Marc";
System.out.println("Who is " + who);
// properties in the classpath
Properties props = new java.util.Properties();
java.net.URL url =
ClassLoader.getSystemResource("context.properties");
props.load(url.openStream());
System.out.println(props);
String xml =
"<HelloWorldProcessRequest
xmlns=\"http://bpel.eurotransplant.nl/HelloWorld\">";
xml = xml + "<input>" + who + "</input>";
xml = xml + "</HelloWorldProcessRequest>";
Locator locator = new Locator("default", "bpel", props);
IDeliveryService deliveryService =
(IDeliveryService)
locator.lookupService(IDeliveryService.SERVICE_NAME);
NormalizedMessage nm = new NormalizedMessage();
nm.addPart("payload", xml);
NormalizedMessage res =
deliveryService.request("HelloWorld", "process", nm);
Map payload = res.getPayload();
System.out.println("BPELProcess HelloWorld executed!<br>");
Element partEl = (Element) payload.get("payload");
System.out.println("The Result was " + XMLHelper.toXML(partEl));
}
catch (Exception e)
{
e.printStackTrace();
}
}
}